【Laravel】Swaggerを使ってAPI設計書を作ってみる
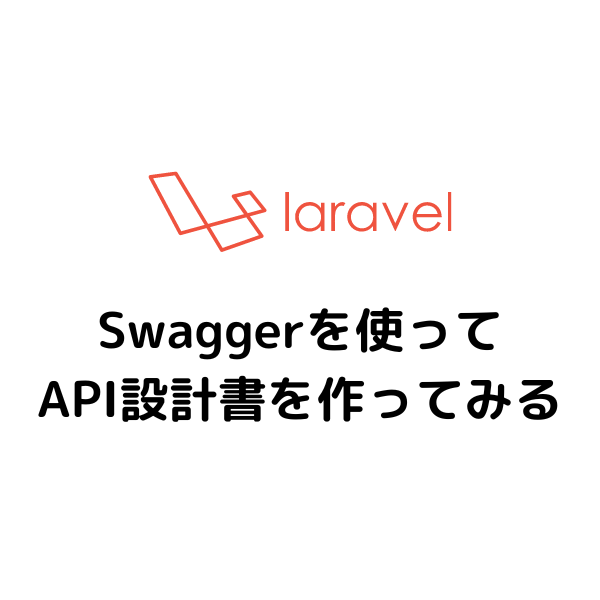
~簡単な自己紹介~
- 嫁と猫3匹と暮らすフルリモートの三十路SE
- ブリッジSE的なポジションを務めることが多く、チーム運営、顧客折衝、ベンダーコントロールが得意
- 投資・節約・副業で資産形成中
- 現在の金融資産は約1,650万円、めざせアッパーマス層(資産3,000万円)
プロジェクトで設計書としてSwaggerを使っています。
私は開発担当なので今までは見るだけですが、今後の為にSwaggerの導入方法を勉強してみました。
大きな開発だと基本設計書に○○テーブルから△△の条件でデータを取得して、取得したデータをループしてどうやって加工するか・・・といったところまで書き起こしてありますが、Swaggerはパラメーター(IN)とレスポンス(OUT)が書いてあるだけなので、設計書というよりも仕様書に近いと感じています。
目次
開発環境
- OS:Windows11
- Docker Desktopインストール済み
- VSCodeインストール済み
下記の記事で作成した開発環境にSwaggerをインストールしていきます。
Swaggerをインストールする
ターミナルを表示する
メニューの表示から、ターミナルを開きます。
ショートカットキーはCtrl + @なので、覚えておくと便利です。
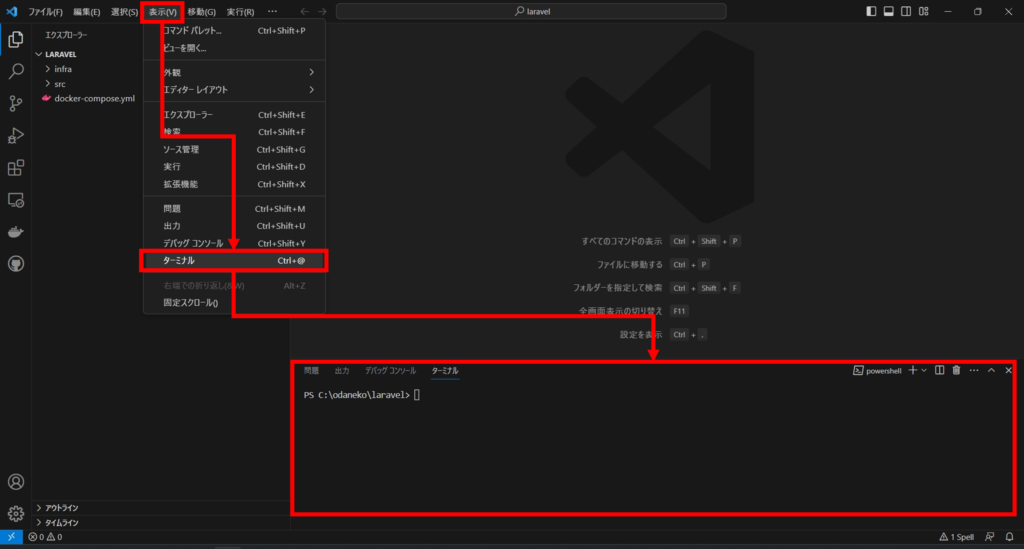
appコンテナに入る
下記のコマンドを実行して、appコンテナに入ります。
docker compose exec app bash
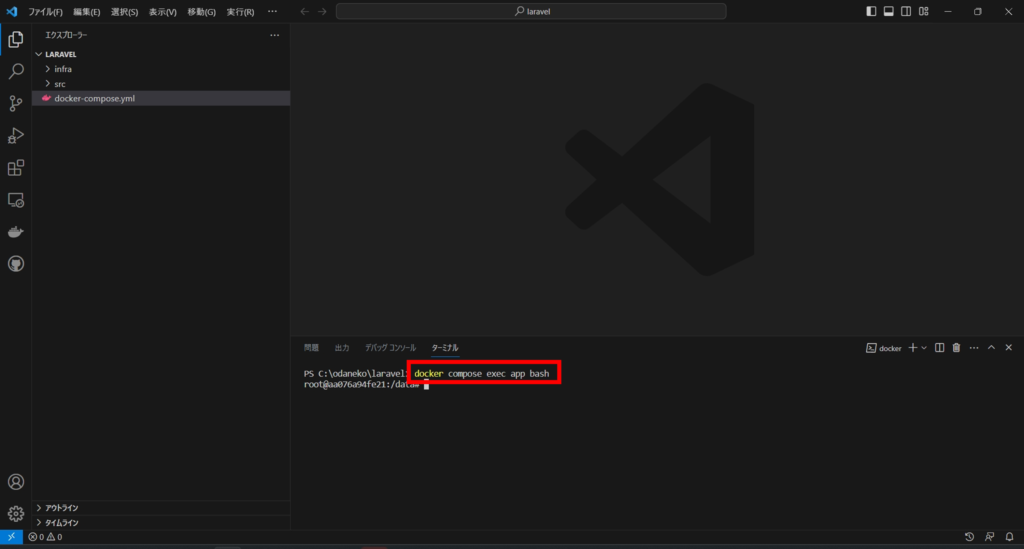
Swaggerをインストールする
下記のコマンドを実行して、Swaggerをインストールします。
composer require "darkaonline/l5-swagger"
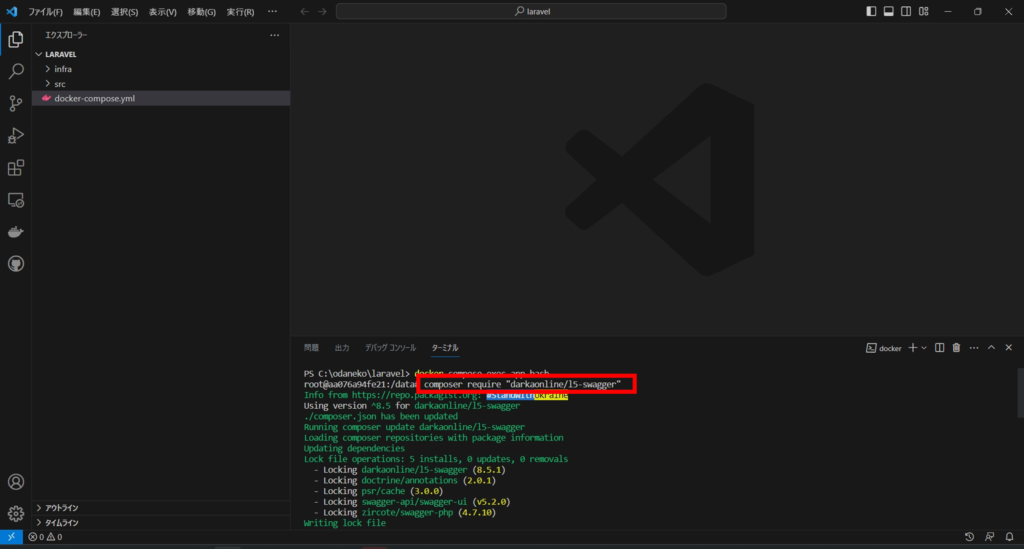
Controller.phpを編集する
Controller.phpに下記の内容を追加します。
use OpenApi\Annotations as OA;
/**
* @OA\Info(
* version="1.0.0",
* title="Swaggerテスト",
* description="API仕様書"
*
* )
*/
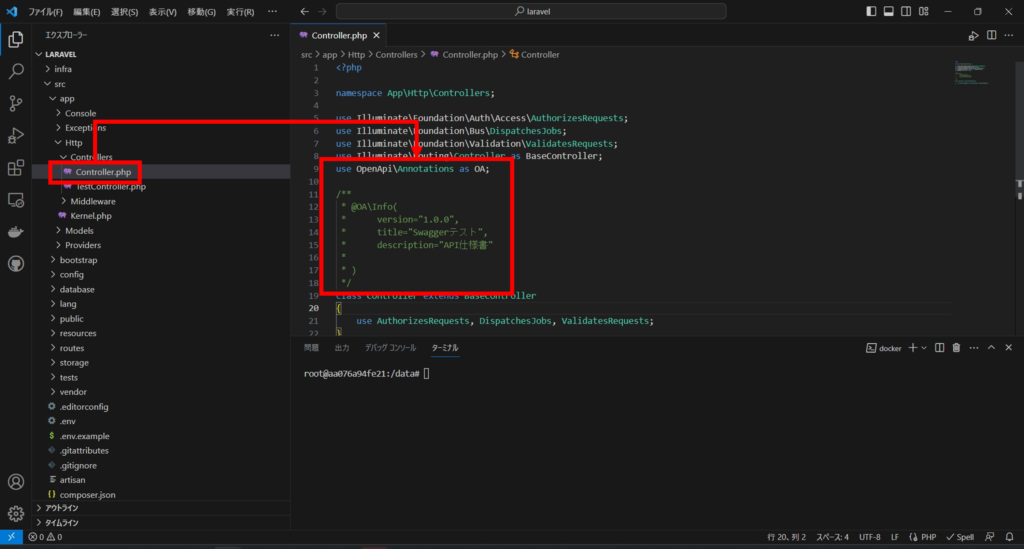
APIのコントローラーを用意する
コマンドでコントローラーを作成する①~部署~
下記のコマンドを実行して、部署APIのコントローラーを作成します。
php artisan make:controller DepartmentController
作成したコントローラークラスに下記の内容を追記します。
少し長いですが、コード右上のバインダーみたいなマークをクリックするとコピーできます。
/**
* @OA\Get(
* path="/api/department/{id}",
* tags={"部署マスタ"},
* summary="ID検索",
*
* @OA\Parameter(
* name="id",
* description="ID",
* in="path",
* required=true,
* @OA\Schema(type="int")
* ),
*
* @OA\Response(
* response="200",
* description="OK",
* @OA\JsonContent(
* type="object",
* example={
* "id":1,
* "name":"システム部"
* },
* @OA\Property(property="id",type="int"),
* @OA\Property(property="name",type="string")
* )
* ),
* @OA\Response(response=400, description="Bad Request"),
* @OA\Response(response=401, description="Unauthorized"),
* @OA\Response(response=403, description="Forbidden"),
* @OA\Response(response=404, description="Not Found"),
* )
*
* Handle the incoming request.
*
* @param \Illuminate\Http\Request $request
*/
public function selectById()
{
}
/**
* @OA\Get(
* path="/api/department",
* tags={"部署マスタ"},
* summary="部署名検索",
*
* @OA\Parameter(
* name="name",
* description="名称",
* in="query",
* required=true,
* @OA\Schema(type="string")
* ),
*
* @OA\Response(
* response="200",
* description="OK",
* @OA\JsonContent(
* type="object",
* example={
* {
* "id":1,
* "name":"システム部"
* },
* {
* "id":2,
* "name":"総務部"
* },
* },
* @OA\Property(property="id",type="int"),
* @OA\Property(property="name",type="string")
* )
* ),
* @OA\Response(response=400, description="Bad Request"),
* @OA\Response(response=401, description="Unauthorized"),
* @OA\Response(response=403, description="Forbidden"),
* @OA\Response(response=404, description="Not Found"),
* )
*
* Handle the incoming request.
*
* @param \Illuminate\Http\Request $request
*/
public function selectByName()
{
}
/**
* @OA\Post(
* path="/api/department",
* tags={"部署マスタ"},
* summary="登録",
*
* @OA\Parameter(
* name="name",
* description="名称",
* in="query",
* required=true,
* @OA\Schema(type="string")
* ),
*
* @OA\Response(
* response="200",
* description="OK",
* @OA\JsonContent(
* type="object",
* example={
* "id":1,
* "name":"システム部"
* },
* @OA\Property(property="id",type="int"),
* @OA\Property(property="name",type="string")
* )
* ),
* @OA\Response(response=400, description="Bad Request"),
* @OA\Response(response=401, description="Unauthorized"),
* @OA\Response(response=403, description="Forbidden"),
* @OA\Response(response=404, description="Not Found"),
* )
*
* Handle the incoming request.
*
* @param \Illuminate\Http\Request $request
*/
public function insert()
{
}
/**
* @OA\Put(
* path="/api/department/{id}",
* tags={"部署マスタ"},
* summary="更新",
*
* @OA\Parameter(
* name="id",
* description="ID",
* in="path",
* required=true,
* @OA\Schema(type="int")
* ),
* @OA\Parameter(
* name="name",
* description="名称",
* in="query",
* required=true,
* @OA\Schema(type="string")
* ),
*
* @OA\Response(
* response="200",
* description="OK",
* @OA\JsonContent(
* type="object",
* example={
* "id":1,
* "name":"システム部"
* },
* @OA\Property(property="id",type="int"),
* @OA\Property(property="name",type="string")
* )
* ),
* @OA\Response(response=400, description="Bad Request"),
* @OA\Response(response=401, description="Unauthorized"),
* @OA\Response(response=403, description="Forbidden"),
* @OA\Response(response=404, description="Not Found"),
* )
*
* Handle the incoming request.
*
* @param \Illuminate\Http\Request $request
*/
public function update()
{
}
/**
* @OA\Delete(
* path="/api/department/{id}",
* tags={"部署マスタ"},
* summary="削除",
*
* @OA\Parameter(
* name="id",
* description="ID",
* in="path",
* required=true,
* @OA\Schema(type="int")
* ),
*
* @OA\Response(
* response="200",
* description="OK",
* ),
* @OA\Response(response=400, description="Bad Request"),
* @OA\Response(response=401, description="Unauthorized"),
* @OA\Response(response=403, description="Forbidden"),
* @OA\Response(response=404, description="Not Found"),
* )
*
* Handle the incoming request.
*
* @param \Illuminate\Http\Request $request
*/
public function delete()
{
}
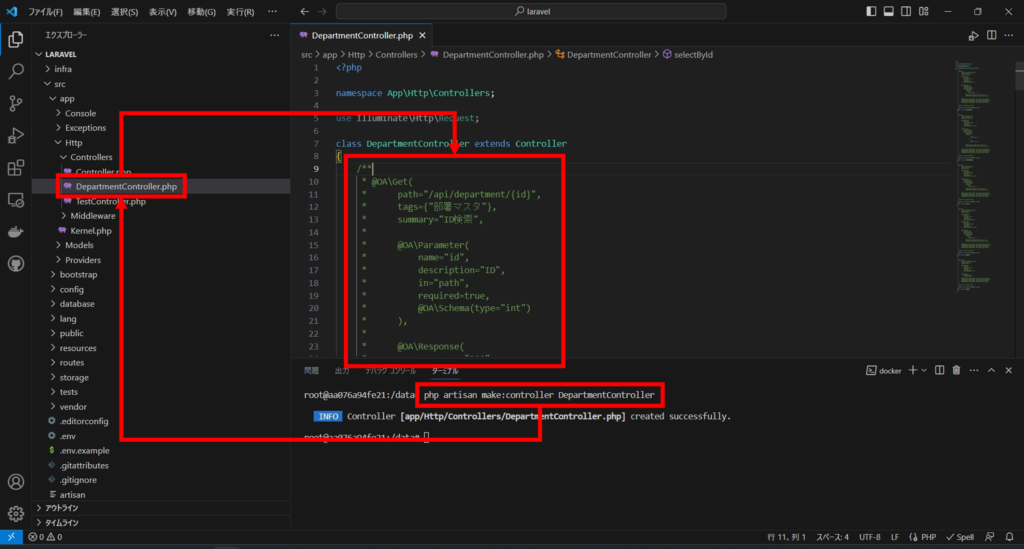
コマンドでコントローラーを作成する②~社員~
下記のコマンドを実行して、社員APIのコントローラーを作成します。
php artisan make:controller EmployeeController
作成したコントローラークラスに下記の内容を追記します。
少し長いですが、コード右上のバインダーみたいなマークをクリックするとコピーできます。
/**
* @OA\Get(
* path="/api/employee/{id}",
* tags={"社員マスタ"},
* summary="ID検索",
*
* @OA\Parameter(
* name="id",
* description="ID",
* in="path",
* required=true,
* @OA\Schema(type="int")
* ),
*
* @OA\Response(
* response="200",
* description="OK",
* @OA\JsonContent(
* type="object",
* example={
* "id":1,
* "name":"東京たろう",
* "department_id":1,
* },
* @OA\Property(property="id",type="int"),
* @OA\Property(property="name",type="string"),
* @OA\Property(property="department_id",type="int"),
* )
* ),
* @OA\Response(response=400, description="Bad Request"),
* @OA\Response(response=401, description="Unauthorized"),
* @OA\Response(response=403, description="Forbidden"),
* @OA\Response(response=404, description="Not Found"),
* )
*
* Handle the incoming request.
*
* @param \Illuminate\Http\Request $request
*/
public function selectById()
{
}
/**
* @OA\Get(
* path="/api/employee",
* tags={"社員マスタ"},
* summary="社員名検索",
*
* @OA\Parameter(
* name="name",
* description="名称",
* in="query",
* required=true,
* @OA\Schema(type="string")
* ),
* @OA\Parameter(
* name="department_id",
* description="部署ID",
* in="query",
* required=false,
* @OA\Schema(type="int")
* ),
*
* @OA\Response(
* response="200",
* description="OK",
* @OA\JsonContent(
* type="object",
* example={
* {
* "id":1,
* "name":"システム部",
* "department_id":1,
* },
* {
* "id":2,
* "name":"総務部",
* "department_id":1,
* },
* },
* @OA\Property(property="id",type="int"),
* @OA\Property(property="name",type="string"),
* @OA\Property(property="department_id",type="int"),
* )
* ),
* @OA\Response(response=400, description="Bad Request"),
* @OA\Response(response=401, description="Unauthorized"),
* @OA\Response(response=403, description="Forbidden"),
* @OA\Response(response=404, description="Not Found"),
* )
*
* Handle the incoming request.
*
* @param \Illuminate\Http\Request $request
*/
public function selectByName()
{
}
/**
* @OA\Post(
* path="/api/employee",
* tags={"社員マスタ"},
* summary="登録",
*
* @OA\Parameter(
* name="name",
* description="名称",
* in="query",
* required=true,
* @OA\Schema(type="string")
* ),
* @OA\Parameter(
* name="department_id",
* description="部署ID",
* in="query",
* required=true,
* @OA\Schema(type="int")
* ),
*
* @OA\Response(
* response="200",
* description="OK",
* @OA\JsonContent(
* type="object",
* example={
* "id":1,
* "name":"システム部",
* "department_id":1,
* },
* @OA\Property(property="id",type="int"),
* @OA\Property(property="name",type="string"),
* @OA\Property(property="department_id",type="int"),
* )
* ),
* @OA\Response(response=400, description="Bad Request"),
* @OA\Response(response=401, description="Unauthorized"),
* @OA\Response(response=403, description="Forbidden"),
* @OA\Response(response=404, description="Not Found"),
* )
*
* Handle the incoming request.
*
* @param \Illuminate\Http\Request $request
*/
public function insert()
{
}
/**
* @OA\Put(
* path="/api/employee/{id}",
* tags={"社員マスタ"},
* summary="更新",
*
* @OA\Parameter(
* name="id",
* description="ID",
* in="path",
* required=true,
* @OA\Schema(type="int")
* ),
* @OA\Parameter(
* name="name",
* description="名称",
* in="query",
* required=false,
* @OA\Schema(type="string")
* ),
* @OA\Parameter(
* name="department_id",
* description="部署ID",
* in="query",
* required=false,
* @OA\Schema(type="int")
* ),
*
* @OA\Response(
* response="200",
* description="OK",
* @OA\JsonContent(
* type="object",
* example={
* "id":1,
* "name":"システム部",
* "department_id":1,
* },
* @OA\Property(property="id",type="int"),
* @OA\Property(property="name",type="string"),
* @OA\Property(property="department_id",type="int"),
* )
* ),
* @OA\Response(response=400, description="Bad Request"),
* @OA\Response(response=401, description="Unauthorized"),
* @OA\Response(response=403, description="Forbidden"),
* @OA\Response(response=404, description="Not Found"),
* )
*
* Handle the incoming request.
*
* @param \Illuminate\Http\Request $request
*/
public function update()
{
}
/**
* @OA\Delete(
* path="/api/employee/{id}",
* tags={"社員マスタ"},
* summary="削除",
*
* @OA\Parameter(
* name="id",
* description="ID",
* in="path",
* required=true,
* @OA\Schema(type="int")
* ),
*
* @OA\Response(
* response="200",
* description="OK",
* ),
* @OA\Response(response=400, description="Bad Request"),
* @OA\Response(response=401, description="Unauthorized"),
* @OA\Response(response=403, description="Forbidden"),
* @OA\Response(response=404, description="Not Found"),
* )
*
* Handle the incoming request.
*
* @param \Illuminate\Http\Request $request
*/
public function delete()
{
}
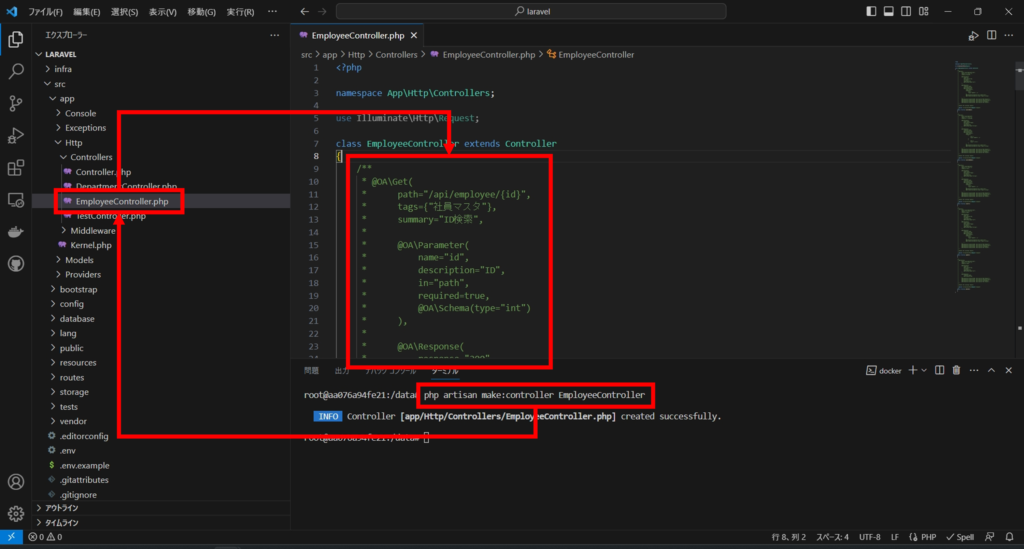
設計書を生成する
設計書を生成する
下記のコマンドを実行して、Swagger(設計書)を生成します。
php artisan l5-swagger:generate
特にエラーなく完了すればOKです。
ブラウザでSwagger(設計書)を確認する
下記のURLを開いて、ブラウザ上でAPI設計書を確認します。
http://localhost:8080/api/documentation
下記のような表示になっていればOKです。
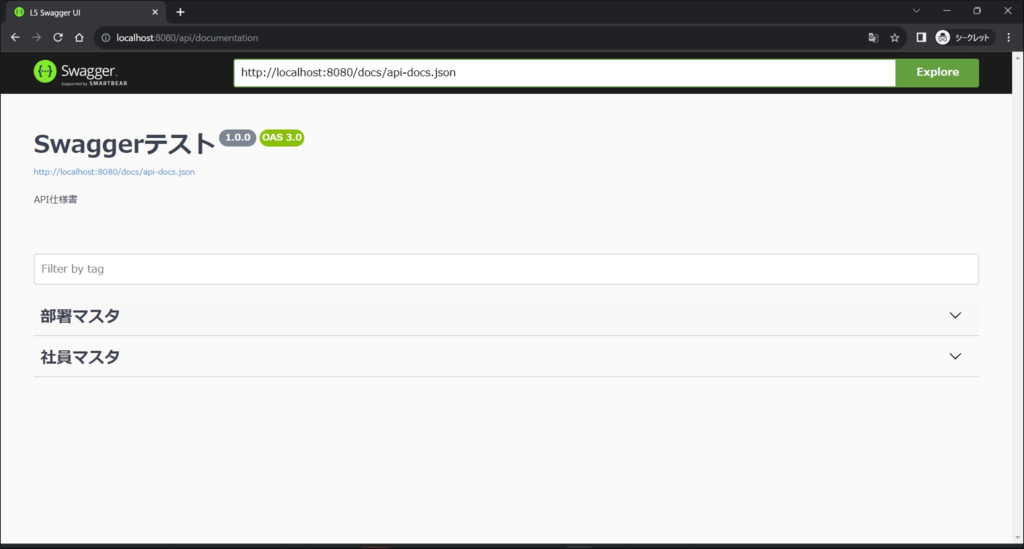
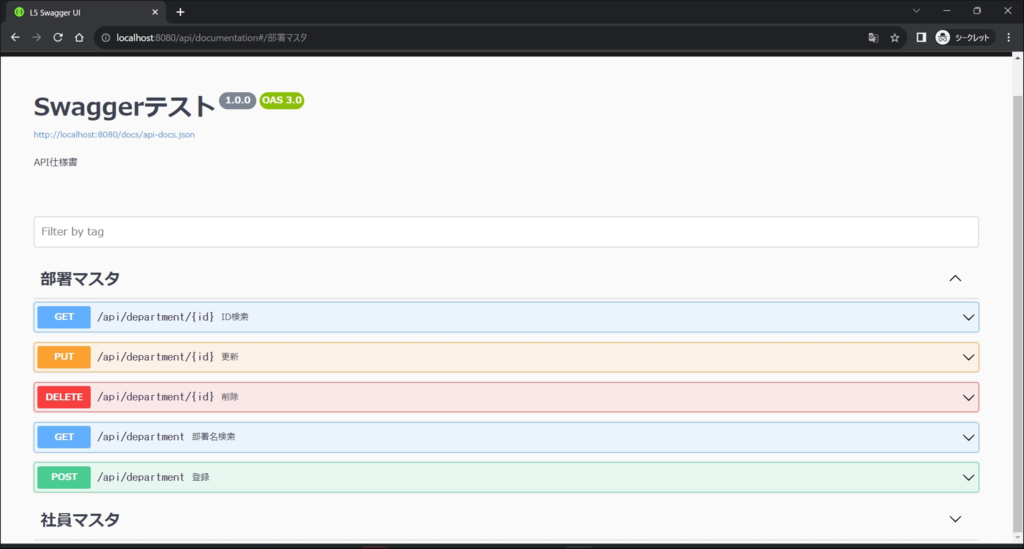
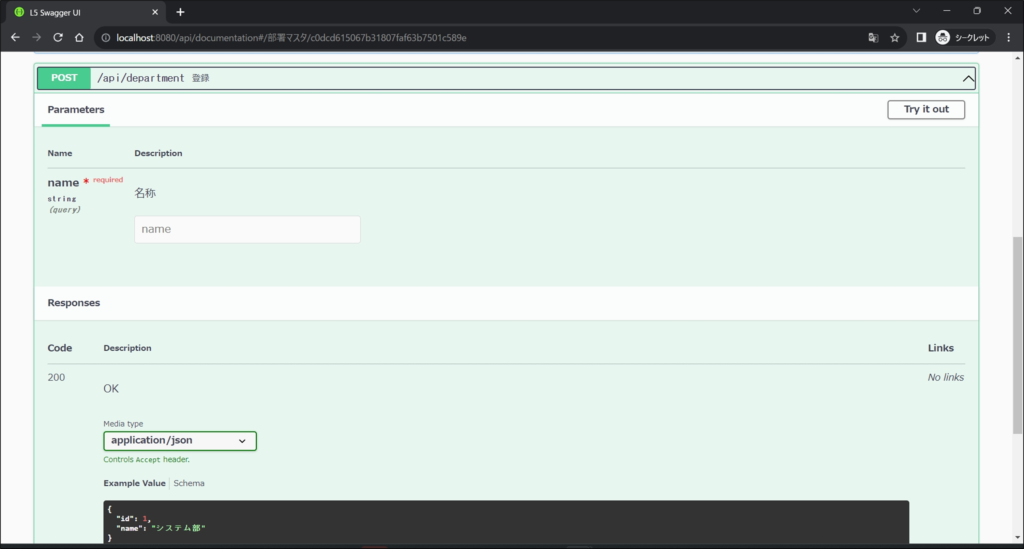
以上になります。
お疲れさまでした。